Today I was attending some time management training but it turned surprisingly interesting. Anyway, it was more about how we function, the concious and subconcious states. Basically, it seems we switch around 40 times to the subconcious state.
Are you fully awake when in the morning you take a shower?
Or does it happen that when you enter the office it clicks to you, how the heck I drove to here?
That's the subconcious baby. It seems the subconcious manages 250 million instructions per minute while the concious just 700... I may be incorrect on these figures but anyway just to emphasize the difference.
I unconciously did a lot of these things... do you ever want to switch to the subconcious state when you want to implement some piece of code?
I do, I put on my ear plugs, listen to some music (I listen to the same music, if I listen to some new album than I switch back to the concious state!), detach from the surroundings and get lost in my coding! That's why I like my job!
I prefer to work in this subconcious state than doing pair programming!
Personally I think I am more productive and deliver better when I work in this state than working in pairs... That's my way of thinking.
Check out this link for more information
Thursday, November 5, 2009
Sunday, November 1, 2009
Removing a workspace from Eclipse
Occasionally one wants to remove a workspace from Eclipse.
This is how one can remove it from launching a deleted workspace.
This is how one can remove it from launching a deleted workspace.
- Close Eclipse if its running
- Browse to the folder /configuration/.settings in the Eclipse installation folder (%ECLIPSE_HOME%)
- Note that .settings is a hidden folder (Ctrl-H in Ubuntu to display hidden files)
- Open the file org.eclipse.ui.ide.prefs. This is where Eclipse stores workspace information.
- Just edit the key named RECENT_WORKSPACES
- Save and restart Eclipse.
Thursday, October 29, 2009
Getting Spring POJOs from external Spring unaware systems
There are cases when one wants to instantiate POJOs from classes that are not Spring aware (like old EJBs).
In such circumstances, one needs to get the Spring application context and retrieve beans from the Spring container.
This is how you do it:
1. Create a class that provides the Spring application context from the container
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
/**
* This class provides an application-wide access to the
* Spring ApplicationContext!
*
* Use AppContext.getApplicationContext() to get access
* to all Spring Beans.
*
*/
public class ApplicationContextProvider implements ApplicationContextAware
{
private static ApplicationContext ctx;
private ApplicationContextProvider(){}
public static ApplicationContext getApplicationContext()
{
return ctx;
}
@Override
public void setApplicationContext(ApplicationContext ctx) throws BeansException
{
this.ctx = ctx;
}
}
2. Wire it in Spring
<bean id="contextApplicationContextProvider" class="com.yournamespace.ApplicationContextProvider"></bean>
3. Get a bean from any other class
SomeBean bean = (SomeBean)ApplicationContextProvider.getApplicationContext().getBean("someBean");
In such circumstances, one needs to get the Spring application context and retrieve beans from the Spring container.
This is how you do it:
1. Create a class that provides the Spring application context from the container
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
/**
* This class provides an application-wide access to the
* Spring ApplicationContext!
*
* Use AppContext.getApplicationContext() to get access
* to all Spring Beans.
*
*/
public class ApplicationContextProvider implements ApplicationContextAware
{
private static ApplicationContext ctx;
private ApplicationContextProvider(){}
public static ApplicationContext getApplicationContext()
{
return ctx;
}
@Override
public void setApplicationContext(ApplicationContext ctx) throws BeansException
{
this.ctx = ctx;
}
}
2. Wire it in Spring
<bean id="contextApplicationContextProvider" class="com.yournamespace.ApplicationContextProvider"></bean>
3. Get a bean from any other class
SomeBean bean = (SomeBean)ApplicationContextProvider.getApplicationContext().getBean("someBean");
Thursday, October 22, 2009
Building an Agile Team
I have read this article with interest regarding building an Agile Team
Very good points, summarizing everything in two words: building an Agile Team, its all about communication and resources... good resources!
But from experience I would like to add the following point:
What about one tries to build an Agile team with the existing resources?
This is the common scenario were one tries to introduce Agile in an existing technical department.
I think Agile\Scrum is all about common sense. Definitely as stated in the article, communication is what makes the success of an Agile team but also the continuous improvement, sprint after sprint.
And I think that's what makes sprint retrospectives very important. From experience, sprint retrospectives are not given the necessary attention. Sometimes, retrospectives are done for the sake of doing them.
A retrospective should be a 'mini-revolution'.
Pigs should openly speak of what is hindering their velocity and how can improve it like:
Very good points, summarizing everything in two words: building an Agile Team, its all about communication and resources... good resources!
But from experience I would like to add the following point:
What about one tries to build an Agile team with the existing resources?
This is the common scenario were one tries to introduce Agile in an existing technical department.
I think Agile\Scrum is all about common sense. Definitely as stated in the article, communication is what makes the success of an Agile team but also the continuous improvement, sprint after sprint.
And I think that's what makes sprint retrospectives very important. From experience, sprint retrospectives are not given the necessary attention. Sometimes, retrospectives are done for the sake of doing them.
A retrospective should be a 'mini-revolution'.
Pigs should openly speak of what is hindering their velocity and how can improve it like:
- Software used for the Agile process
- External forces interfering the team
- Lacking of particular resources
- Problems with the current architecture, framework used...and so on.
Monday, September 14, 2009
ASP.Net Setting Text for a Textbox with TextMode = Password
When one sets the Text property in a TextBox with TextMode = Password, the default behaviour is to render the TextBox empty.
Basically for security reasons and that's the normal and correct behaviour.
But and there is always a BUTT, one may want to set the Text in a TextBox with TextMode = Password.
The way to do it is as follows:
txtPwd.Attributes.Add("value", customer.Password);
Basically for security reasons and that's the normal and correct behaviour.
But and there is always a BUTT, one may want to set the Text in a TextBox with TextMode = Password.
The way to do it is as follows:
txtPwd.Attributes.Add("value", customer.Password);
Tuesday, September 8, 2009
Call JQuery code from ASP.Net server side
A little trick to call JQuery code from the server side.
A typical example is that when one wants to show different panels of a wizard or when a form is submitted successfully, one wants to show 'Congratulations' page.
Basically one just needs to inject the Javascript code in the server side code and JQuery will execute it once it is in control.
Like the following code snippet:
StringBuilder sb = new StringBuilder();
sb.AppendLine("$(document).ready(function() {");
sb.AppendLine("showForm();");
sb.AppendLine(" });");
A typical example is that when one wants to show different panels of a wizard or when a form is submitted successfully, one wants to show 'Congratulations' page.
Basically one just needs to inject the Javascript code in the server side code and JQuery will execute it once it is in control.
Like the following code snippet:
StringBuilder sb = new StringBuilder();
sb.AppendLine("$(document).ready(function() {");
sb.AppendLine("showForm();");
sb.AppendLine(" });");
Page.ClientScript.RegisterClientScriptBlock(typeof(Page),Guid.NewGuid().ToString(), sb.ToString(), true);
Sidenote, the JQuery document ready function can be in an external javascript file. The above will still work.Thursday, September 3, 2009
Tuesday, August 25, 2009
SQL Server Empty All Tables
The other day I had to empty a database from all its data.
I found this link which did my job. It has a little stored procedure that I created in my database and just executed it.
I slightly modified the stored procedure to exclude some reference tables.
What I recommend after emptying the database is to compact it.
In SQL Server Management Studio one can select the database, right click choose
Tasks -> Shrink -> Database
That's all!
I found this link which did my job. It has a little stored procedure that I created in my database and just executed it.
I slightly modified the stored procedure to exclude some reference tables.
What I recommend after emptying the database is to compact it.
In SQL Server Management Studio one can select the database, right click choose
Tasks -> Shrink -> Database
That's all!
Friday, August 21, 2009
JAX-WS "Two classes have the same XML type name"
When generating the Java classes using wsgen from a WSDL I was getting the following error:
Two classes have the same XML type name "{http://myservice/}foo". Use @XmlType.name and @XmlType.namespace to assign different names to them.
this problem is related to the following location:
As suggested by the exception I tried changing @XmlType but that was fine for me.
This link offers the same solution and it also suggests to change Response classes which I think its an extreme solution.
Anyway in my case the problem was that I generated the classes and I wanted to change the package name.
Everything worked fine, compiled and the webservice deployed successfully.
When viewing the WSDL I got the above error.
In my case the solution was just to update the new namespace in the Webmethod attribute.
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "foo", targetNamespace = "http://fooservice/", className = "com.foo.service.generated.foo")
@ResponseWrapper(localName = "fooResponse", targetNamespace = "http://fooservice/", className = "com.foo.service.generated.FooResponse")
The attribute className had to be updated :(
Two classes have the same XML type name "{http://myservice/}foo". Use @XmlType.name and @XmlType.namespace to assign different names to them.
this problem is related to the following location:
As suggested by the exception I tried changing @XmlType but that was fine for me.
This link offers the same solution and it also suggests to change Response classes which I think its an extreme solution.
Anyway in my case the problem was that I generated the classes and I wanted to change the package name.
Everything worked fine, compiled and the webservice deployed successfully.
When viewing the WSDL I got the above error.
In my case the solution was just to update the new namespace in the Webmethod attribute.
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "foo", targetNamespace = "http://fooservice/", className = "com.foo.service.generated.foo")
@ResponseWrapper(localName = "fooResponse", targetNamespace = "http://fooservice/", className = "com.foo.service.generated.FooResponse")
The attribute className had to be updated :(
Tuesday, August 4, 2009
Java FileWriter UTF-8 encoding
Not possible to specify encoding for a FileWriter object.
The documentation states that FileWriter is a convenient class depending on the underlying platform.
The easiest solution is to use an OutputStreamWriter. Something as follows, in my case I am exporting to CSVWriter:
FileOutputStream fos = new FileOutputStream(new File(fileName));
OutputStreamWriter osw = new OutputStreamWriter(fos, "UTF-8");
CSVWriter writer = new CSVWriter(osw, CSV_DELIMITER);
The documentation states that FileWriter is a convenient class depending on the underlying platform.
The easiest solution is to use an OutputStreamWriter. Something as follows, in my case I am exporting to CSVWriter:
FileOutputStream fos = new FileOutputStream(new File(fileName));
OutputStreamWriter osw = new OutputStreamWriter(fos, "UTF-8");
CSVWriter writer = new CSVWriter(osw, CSV_DELIMITER);
Tuesday, July 21, 2009
HttpClient uploading large files
When using HTTPClient to upload large files I was getting the following exception:
org.apache.commons.httpclient.HttpMethodDirector executeWithRetry
INFO: I/O exception (java.net.SocketException) caught when processing request: Software caused connection abort: socket write error
org.apache.commons.httpclient.HttpMethodDirector executeWithRetry
INFO: Retrying request
java.lang.Exception: Unbuffered entity enclosing request can not be repeated.
I had the following code:
PutMethod method = new PutMethod(url + "/" + file.getName());
RequestEntity requestEntity = new InputStreamRequestEntity(new FileInputStream(file));
method.setRequestEntity(requestEntity);
client.executeMethod(method);
I replaced the code as follows:
String filename = url + "/" + file.getName();
PutMethod method = new PutMethod(filename);
method.getParams().setBooleanParameter(HttpMethodParams.USE_EXPECT_CONTINUE, true);
Part[] parts = { new FilePart(file.getName(), file) };
method.setRequestEntity(new MultipartRequestEntity(parts, method.getParams()));
client.executeMethod(method);
In my case I am uploading (PUTMETHOD) to a WebDAV folder. But I noticed that this issue is happening for other protocols too.
Basically using MultiPart and setting the parameter USE_EXPECT_CONTINUE to true solved the problem.
The problem is due to the authentication. When the post has a big file it opens the transfer multiple times. And I guess the socket will be closed or not authenticated when there is the second transfer.
org.apache.commons.httpclient.HttpMethodDirector executeWithRetry
INFO: I/O exception (java.net.SocketException) caught when processing request: Software caused connection abort: socket write error
org.apache.commons.httpclient.HttpMethodDirector executeWithRetry
INFO: Retrying request
java.lang.Exception: Unbuffered entity enclosing request can not be repeated.
I had the following code:
PutMethod method = new PutMethod(url + "/" + file.getName());
RequestEntity requestEntity = new InputStreamRequestEntity(new FileInputStream(file));
method.setRequestEntity(requestEntity);
client.executeMethod(method);
I replaced the code as follows:
String filename = url + "/" + file.getName();
PutMethod method = new PutMethod(filename);
method.getParams().setBooleanParameter(HttpMethodParams.USE_EXPECT_CONTINUE, true);
Part[] parts = { new FilePart(file.getName(), file) };
method.setRequestEntity(new MultipartRequestEntity(parts, method.getParams()));
client.executeMethod(method);
In my case I am uploading (PUTMETHOD) to a WebDAV folder. But I noticed that this issue is happening for other protocols too.
Basically using MultiPart and setting the parameter USE_EXPECT_CONTINUE to true solved the problem.
The problem is due to the authentication. When the post has a big file it opens the transfer multiple times. And I guess the socket will be closed or not authenticated when there is the second transfer.
Monday, July 20, 2009
Find empty tables in SQL Server 2005
To find all the empty tables in your SQL Server 2005 you can use the following query:
SELECT o.name, sum(s.row_count)
FROM sys.objects o
JOIN sys.dm_db_partition_stats s
ON o.object_id=s.object_id
WHERE o.type='U'
GROUP BY o.name
HAVING sum(s.row_count) = 0
ORDER BY sum(s.row_count) desc
where 'U' stands for User Table
Just in case one wants to find out if a table is used in stored procedures, triggers and so on... one can use the following SQL:
SELECT o.name,o.xtype,m.definition
FROM sys.sql_modules m
INNER JOIN sysobjects o ON m.object_id=o.id
WHERE [definition] LIKE '%TABLE_NAME%'
SELECT o.name, sum(s.row_count)
FROM sys.objects o
JOIN sys.dm_db_partition_stats s
ON o.object_id=s.object_id
WHERE o.type='U'
GROUP BY o.name
HAVING sum(s.row_count) = 0
ORDER BY sum(s.row_count) desc
where 'U' stands for User Table
Just in case one wants to find out if a table is used in stored procedures, triggers and so on... one can use the following SQL:
SELECT o.name,o.xtype,m.definition
FROM sys.sql_modules m
INNER JOIN sysobjects o ON m.object_id=o.id
WHERE [definition] LIKE '%TABLE_NAME%'
Thursday, July 16, 2009
WebDAV problems
If you have problems with WebDAV this is a great link to troubleshoot WebDAV problems
Wednesday, July 15, 2009
Problem with AspectJ and Eclipse using maven
Having problems with AspectJ dependencies missing in the generated Eclipse .classpath file (mvn eclipse:eclipse) . The solution is to set:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-eclipse-plugin</artifactId>
<configuration>
<ajdtVersion>none</ajdtVersion>
</configuration>
</plugin>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>aspectj-maven-plugin</artifactId>
<configuration>
<aspectLibraries>
<aspectLibrary>
<groupId>org.thirdparty</groupId>
<artifactId>jar-containing-external-aspects</artifactId>
</aspectLibrary>
</aspectLibraries>
<weaveDependencies>
<weaveDependency>
<groupId>org.mycompany</groupId>
<artifactId>jar-to-weave-with-aspects-in-this-library</artifactId>
</weaveDependency>
</weaveDependencies>
</configuration>
</plugin>
ajdtVersion to "none"Here is how to set it in the POM file:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-eclipse-plugin</artifactId>
<configuration>
<ajdtVersion>none</ajdtVersion>
</configuration>
</plugin>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>aspectj-maven-plugin</artifactId>
<configuration>
<aspectLibraries>
<aspectLibrary>
<groupId>org.thirdparty</groupId>
<artifactId>jar-containing-external-aspects</artifactId>
</aspectLibrary>
</aspectLibraries>
<weaveDependencies>
<weaveDependency>
<groupId>org.mycompany</groupId>
<artifactId>jar-to-weave-with-aspects-in-this-library</artifactId>
</weaveDependency>
</weaveDependencies>
</configuration>
</plugin>
Friday, June 5, 2009
Glassfish domain startup
Recently when starting one of the domains on GlassFish I was getting a whole bunch of exceptions.
The first exception was the following:
"IOP00410216: (COMM_FAILURE) Unable to create IIOP listener on the specified host/port: all interfaces/2555"
org.omg.CORBA.COMM_FAILURE: vmcid: SUN minor code: 216 completed: No
Googled around and I didn't find a proper solution.
Quick fix for me was to change the IIOP port in /config/domain.xml
Look for iiop-listener and just change the port.
Weird thing is that I did a netstat and the original port was not taken.
Anyway this was the quick fix for me.
The first exception was the following:
"IOP00410216: (COMM_FAILURE) Unable to create IIOP listener on the specified host/port: all interfaces/2555"
org.omg.CORBA.COMM_FAILURE: vmcid: SUN minor code: 216 completed: No
Googled around and I didn't find a proper solution.
Quick fix for me was to change the IIOP port in /config/domain.xml
Look for iiop-listener and just change the port.
Weird thing is that I did a netstat and the original port was not taken.
Anyway this was the quick fix for me.
Monday, May 18, 2009
Google maps API key generation issue
When deploying a web application from STAGE to LIVE I got the following message when trying to use the Google maps API.
I went to generate a Google map key from here as I did for the local and STAGE deploy.
Unfortunately the new key didn't work and I re-generated the key half a dozen times with no success.
Anyway, I diverted my attention to something else and after a couple of hours went back to this issue and miracolously the page came up without the above message.
But I don't believe in miracles... so what have happened I have no idea?
My two cents on this is that the DNS change didn't propogate everywhere until I came back to look again into the problem.
Any other solutions are welcome!
UPDATE: Could be it was a proxy issue and the page was cached somewhere that's the reason behind the delay most probably!
Wednesday, May 13, 2009
Java Open CMS Comparison
There are a bunch of commercial and open source CMS out there.
Which is the best?
As I see it I don't want to bend over for the CMS to work! But the CMS has to work for me.
Functionality I am mainly looking for are the following:
I have used extensively Polopoly... I think its ideal for newspaper type content. Manages nicely translation and entities but lacks on the whizzy content.
On a smaller scale there is Blandware At Leap, similar to Polopoly. Nice approach but personally I think the business logic is tightly coupled with the CMS.
I had a look at the online demos for the rest of the CMSs in the above list.
I liked DotCMS... I will try to install it and give it a go....Will keep you posted!
Any other suitable CMS to add to my list?
Which is the best?
As I see it I don't want to bend over for the CMS to work! But the CMS has to work for me.
Functionality I am mainly looking for are the following:
- 'whizzy' way to modify content.
- Manage entities like categories, products... as proper entities not as documents
- Easy way to manage translation
I have used extensively Polopoly... I think its ideal for newspaper type content. Manages nicely translation and entities but lacks on the whizzy content.
On a smaller scale there is Blandware At Leap, similar to Polopoly. Nice approach but personally I think the business logic is tightly coupled with the CMS.
I had a look at the online demos for the rest of the CMSs in the above list.
I liked DotCMS... I will try to install it and give it a go....Will keep you posted!
Any other suitable CMS to add to my list?
SQL Server performance
When having a website which is under performing one of the bottlenecks could be the database.
Several reasons, database schema not correctly configured, too many calls to the database...
Normally I take this approach:
I switch on the SQL Profiler and look at the queries being sent to the database. I mimic online traffic by using tools like JMeter.
From the Profiler one can easily determine queries that take long and tackle those queries by adding any necessary indices, removing any fields that are not used and so on.
Also, I try to group calls to the database to avoid expensive DB trips.
Additionally one can have two connection pools... For Selects one may use a ReadOnly pool. This will perform queries much faster.
This link has some good tips to look at SQL Performance
Several reasons, database schema not correctly configured, too many calls to the database...
Normally I take this approach:
I switch on the SQL Profiler and look at the queries being sent to the database. I mimic online traffic by using tools like JMeter.
From the Profiler one can easily determine queries that take long and tackle those queries by adding any necessary indices, removing any fields that are not used and so on.
Also, I try to group calls to the database to avoid expensive DB trips.
Additionally one can have two connection pools... For Selects one may use a ReadOnly pool. This will perform queries much faster.
This link has some good tips to look at SQL Performance
Thursday, May 7, 2009
Automated testing with Hudson and Selenium
We use Hudson for continuous integration. I prefer Hudson from CruiseControl since its more user-friendly and one can easily add projects through the easy-to-use GUI instead of manually manipulating XML files.
Selenium is great framework to create automated tests.
My purpose was to automate the automated tests and add them to our build process so we have 'full circle'.
I created a little Java project and an ANT script where one can record tests using the Selenium IDE and adds these tests to our test suite. Test results are displayed using TestNG. If anyone is interested I can send him the test project I created.
My only problem was how to get the Selenium Server running when starting the job through Hudson.
My first attempt was an ANT as the following one:
<target name="start-selenium-server">
<java timeout="300000" fork="true" jar="${ws.home}/selenium-server-1.0-beta-2/selenium-server.jar">
<arg line="-port 4444">
<arg line="'-firefoxProfileTemplate">
</arg>
</arg>
</java>
</target>
While this approach worked fine when running through a command window, unfortunately the fork=true didn't work properly when launched as an ANT build step from Hudson. Hudson waited until the process timed out and didn't start the Selenium Server on a separate JVM.
My second attempt was to have the Selenium Service running as a Windows Service. This approach worked great!
I used Java Service Wrapper
One can easily follow the instructions here to set up JSW.
But this is how I have set up the wrapper.conf file:
Selenium is great framework to create automated tests.
My purpose was to automate the automated tests and add them to our build process so we have 'full circle'.
I created a little Java project and an ANT script where one can record tests using the Selenium IDE and adds these tests to our test suite. Test results are displayed using TestNG. If anyone is interested I can send him the test project I created.
My only problem was how to get the Selenium Server running when starting the job through Hudson.
My first attempt was an ANT as the following one:
<target name="start-selenium-server">
<java timeout="300000" fork="true" jar="${ws.home}/selenium-server-1.0-beta-2/selenium-server.jar">
<arg line="-port 4444">
<arg line="'-firefoxProfileTemplate">
</arg>
</arg>
</java>
</target>
While this approach worked fine when running through a command window, unfortunately the fork=true didn't work properly when launched as an ANT build step from Hudson. Hudson waited until the process timed out and didn't start the Selenium Server on a separate JVM.
My second attempt was to have the Selenium Service running as a Windows Service. This approach worked great!
I used Java Service Wrapper
One can easily follow the instructions here to set up JSW.
But this is how I have set up the wrapper.conf file:
# Java Application wrapper.java.command=%JAVA_HOME%/bin/java # Java Main class. This class must implement the WrapperListener interface # or guarantee that the WrapperManager class is initialized. Helper # classes are provided to do this for you. See the Integration section # of the documentation for details. wrapper.java.mainclass=org.tanukisoftware.wrapper.WrapperSimpleApp # Java Classpath (include wrapper.jar) Add class path elements as # needed starting from 1 wrapper.java.classpath.1=../lib/wrapper.jar wrapper.java.classpath.2=/selenium-server-1.0-beta-2/selenium-server.jar wrapper.java.classpath.3=/selenium-server-1.0-beta-2/selenium-server-coreless.jar wrapper.java.classpath.4=/selenium-server-1.0-beta-2/selenium-server-sources.jar wrapper.java.classpath.5=/selenium-server-1.0-beta-2/selenium-server-tests.jar wrapper.java.classpath.6=/selenium-server-1.0-beta-2/selenium-server-test-sources.jar # Java Library Path (location of Wrapper.DLL or libwrapper.so) wrapper.java.library.path.1=../lib # Java Additional Parameters # Log file to use for wrapper output logging. wrapper.logfile=../logs/wrapper.log wrapper.commandfile=./dump.command wrapper.ntservice.console=true # Initial Java Heap Size (in MB) #wrapper.java.initmemory=3 # Maximum Java Heap Size (in MB) #wrapper.java.maxmemory=64 # Application parameters. Add parameters as needed starting from 1 wrapper.app.parameter.1=org.openqa.selenium.server.SeleniumServer wrapper.app.parameter.2=-port 4444 wrapper.app.parameter.3=-firefoxProfileTemplate "C:\firefoxProfile" #Log Level wrapper.console.loglevel=DEBUG wrapper.logfile.loglevel=DEBUG #******************************************************************** # Wrapper Windows Properties #******************************************************************** # Title to use when running as a console wrapper.console.title=SeleniumService #******************************************************************** # Wrapper Windows NT/2000/XP Service Properties #******************************************************************** # WARNING - Do not modify any of these properties when an application # using this configuration file has been installed as a service. # Please uninstall the service before modifying this section. The # service can then be reinstalled. # Name of the service wrapper.ntservice.name=SeleniumService # Display name of the service wrapper.ntservice.displayname=SeleniumService # Description of the service wrapper.ntservice.description=SeleniumService # Service dependencies. Add dependencies as needed starting from 1 wrapper.ntservice.dependency.1= # Mode in which the service is installed. AUTO_START or DEMAND_START wrapper.ntservice.starttype=AUTO_START # Allow the service to interact with the desktop. wrapper.ntservice.interactive=true
Sunday, May 3, 2009
Agilo for scrum
Agilo for Scrum is an open source, web-based tool to support the Scrum process. It is developed and maintained by agile42, and supports Scrum Teams, Scrum Master, Product Owner and the main ceremonies and artifacts (copied from wikipedia ;) ).
It's free that's what makes appealing compared to Rally and ScrumWorks
Agilo also offers online hosting for your projects.
What's not stated in the website is what a tremendous task is to install this software. I usually give my thumbs down for a software that makes it difficult for me to work with. If its difficult to install, imagine the software itself to use it or the customer support.
Anyway I thought I'll give it a shot... my other opinion regarding open source is that they make it difficult or documentation sucks so you buy their services either consultancy or hosting... Nothing against Agile42 but across the years this is my experience with OSS.
In favour of Agilo, I have to state that I never used Python before. Anyway I found this link which made the installation less painful.
I just need to add the following points or make it more clear to round up what's nicely described in the stated link.
1. Agilo needs Trac to work with and in turn Trac works with Python 2.5. My first attempt before finding the above link was to install the latest version of Python :(
2. I had to install Setuptools to get it working.
3. Adding a user to trac
C:\Python25\Scripts>trac-digest.py -u username -p password >> "C:\Program Files\
Apache Software Foundation\Apache2.2\htdocs\trac\passwords.txt"
Add to administrators
C:\Python25\Scripts>trac-admin.exe "C:\Program Files\Apache Software Foundation\
Apache2.2\htdocs\trac" permission add maab TRAC_ADMIN
3. You can test the installation of trac before setting up Apache with the following command:
C:\Python25\Scripts>tracd-script.py -p 8000 --auth=trac,"C:\Program Files\Apache
Software Foundation\Apache2.2\htdocs\trac\passwords.txt",trac "C:\Program Files
\Apache Software Foundation\Apache2.2\htdocs\trac"
4. This is how I configured Apache
LoadModule python_module modules/mod_python.so
SetHandler mod_python
PythonInterpreter main_interpreter
PythonHandler trac.web.modpython_frontend
PythonOption TracEnv "C:/Program Files/Apache Software Foundation/Apache2.2/htdocs/trac"
PythonOption TracUriRoot /trac
That's all, good luck!
I haven't used the software extensively so far but it looks promising.
It's free that's what makes appealing compared to Rally and ScrumWorks
Agilo also offers online hosting for your projects.
What's not stated in the website is what a tremendous task is to install this software. I usually give my thumbs down for a software that makes it difficult for me to work with. If its difficult to install, imagine the software itself to use it or the customer support.
Anyway I thought I'll give it a shot... my other opinion regarding open source is that they make it difficult or documentation sucks so you buy their services either consultancy or hosting... Nothing against Agile42 but across the years this is my experience with OSS.
In favour of Agilo, I have to state that I never used Python before. Anyway I found this link which made the installation less painful.
I just need to add the following points or make it more clear to round up what's nicely described in the stated link.
1. Agilo needs Trac to work with and in turn Trac works with Python 2.5. My first attempt before finding the above link was to install the latest version of Python :(
2. I had to install Setuptools to get it working.
3. Adding a user to trac
C:\Python25\Scripts>trac-digest.py -u username -p password >> "C:\Program Files\
Apache Software Foundation\Apache2.2\htdocs\trac\passwords.txt"
Add to administrators
C:\Python25\Scripts>trac-admin.exe "C:\Program Files\Apache Software Foundation\
Apache2.2\htdocs\trac" permission add maab TRAC_ADMIN
3. You can test the installation of trac before setting up Apache with the following command:
C:\Python25\Scripts>tracd-script.py -p 8000 --auth=trac,"C:\Program Files\Apache
Software Foundation\Apache2.2\htdocs\trac\passwords.txt",trac "C:\Program Files
\Apache Software Foundation\Apache2.2\htdocs\trac"
4. This is how I configured Apache
LoadModule python_module modules/mod_python.so
SetHandler mod_python
PythonInterpreter main_interpreter
PythonHandler trac.web.modpython_frontend
PythonOption TracEnv "C:/Program Files/Apache Software Foundation/Apache2.2/htdocs/trac"
PythonOption TracUriRoot /trac
That's all, good luck!
I haven't used the software extensively so far but it looks promising.
Friday, April 17, 2009
Glassfish quickies
Here are two quickies for Glassfish.
Quickie 1: How to create a new domain.
where --adminport is the port number for the administration GUI
and --savemasterpassword tells GlassFish to save the master password. It will make it easier then to create a service.
See Also
To see the full syntax and options of the command, type asadmin create-domain --help at the command line.
Quickie 2: Setting GlassFish as a Windows Service
When running GlassFish on a Windows box one has to install it as a service so GlassFish keeps running once you log off.
Here how you do it:
1. Download GlassfishSvc.jar
2. Copy your file to your GlassFish installation and cd to this folder
3. Run the following command:
where -i is to install, -u to uninstall.
See Also
More info here
Quickie 1: How to create a new domain.
asadmin create-domain --adminport 4949 --savemasterpassword true mydomain
and --savemasterpassword tells GlassFish to save the master password. It will make it easier then to create a service.
See Also
To see the full syntax and options of the command, type asadmin create-domain --help at the command line.
Quickie 2: Setting GlassFish as a Windows Service
When running GlassFish on a Windows box one has to install it as a service so GlassFish keeps running once you log off.
Here how you do it:
1. Download GlassfishSvc.jar
2. Copy your file to your GlassFish installation and cd to this folder
3. Run the following command:
C:\Program Files\Sun\GlassfishV2>java -jar GlassfishSvc.jar -i -n ServiceName -m DomainName
See Also
More info here
Thursday, April 16, 2009
Adding users to SVN and setting SVN with Apache
A quickie... to add users in SVN running under Apache, this is the syntax for Windows:
htpasswd D:\Repositories\svn-auth-file jack
You will be prompted as follows:
New password: *******
Re-type new password: *******
Adding password for user jack
Of course when setting up SVN with Apache the svn-auth-file is created.
One can set SVN with Apache as follows:
Copy the the following modules in Apache modules folder:
mod_dav_svn.so, mod_authz_svn.so
Modify the httpd.conf, by adding the following:
# Subversion modules
LoadModule dav_svn_module modules/mod_dav_svn.so
LoadModule authz_svn_module modules/mod_authz_svn.so
htpasswd D:\Repositories\svn-auth-file jack
You will be prompted as follows:
New password: *******
Re-type new password: *******
Adding password for user jack
Of course when setting up SVN with Apache the svn-auth-file is created.
One can set SVN with Apache as follows:
Copy the the following modules in Apache modules folder:
mod_dav_svn.so, mod_authz_svn.so
Modify the httpd.conf, by adding the following:
# Subversion modules
LoadModule dav_svn_module modules/mod_dav_svn.so
LoadModule authz_svn_module modules/mod_authz_svn.so
<location>
DAV svn
SVNPath "C:/Repository/repositoryname"
AuthType Basic
AuthName "Subversion Casino repository"
AuthUserFile "C:/Repository/svn-auth-file"
Require valid-user
<location>
DAV svn
SVNPath "C:/Repository/repositoryname"
AuthType Basic
AuthName "Subversion Casino repository"
AuthUserFile "C:/Repository/svn-auth-file"
Require valid-user
<location>
Sunday, April 12, 2009
Simple Castor unmarshalling example
Castor is great framework to provide Java to XML binding and vice versa. In this little post I am going to show how to unmarshall (marshall-out) an XML file to Java objects.
My sample XML is the following snippet:
Its the Google sitemap.xml. I would like to iterate over the urls in this file to check that there are no errors (no 404s, 500s) in this URL list.
These are the steps to get Java objects in your code representing the above XML.
1. Download Castor from here
2. Create an XSD file for the above XML (there are online schema generator like HIT software
3. Generate Java objects from the schema using an ANT task
4. Write a simple test case to test the code generation:
public void testCastorMarshallingOut() throws FileNotFoundException, MarshalException, ValidationException {
FileReader reader = new FileReader(Settings.getInstance("sitemap"));
Urlset urls = Urlset.unmarshal(reader); assertNotNull(urls);
assertEquals(urls.getUrlCount(), 35);
for(int i = 0; i <>
org.castor.sitemap.Url theUrl = urls.getUrl(i);
String currentUrl = theUrl.getLoc().getContent();
System.out.print(currentUrl); assertNotNull(currentUrl);
}
}
P.S. I had a problem with a required attribute. It seems Castor was not mapping correctly xsi:schemaLocation attribute. In my case I modified the generated XSD, still looking at this issue.
My sample XML is the following snippet:
Its the Google sitemap.xml. I would like to iterate over the urls in this file to check that there are no errors (no 404s, 500s) in this URL list.
<?xml version="1.0" encoding="UTF-8"?>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.google.com/schemas/sitemap/0.84 http://www.sitemaps.org/schemas/sitemap/0.9/sitemap.xsd">
<url><loc>http://www.mysite.com/</loc><lastmod>2009-04-09T18:33:21+00:00</lastmod><changefreq>daily</changefreq><priority>1.00</priority></url>
<url><loc>http://www.mysite.com/register.jsp</loc><lastmod>2009-04-09T18:33:18+00:00</lastmod><changefreq>daily</changefreq><priority>0.50</priority></url>
</urlset>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.google.com/schemas/sitemap/0.84 http://www.sitemaps.org/schemas/sitemap/0.9/sitemap.xsd">
<url><loc>http://www.mysite.com/</loc><lastmod>2009-04-09T18:33:21+00:00</lastmod><changefreq>daily</changefreq><priority>1.00</priority></url>
<url><loc>http://www.mysite.com/register.jsp</loc><lastmod>2009-04-09T18:33:18+00:00</lastmod><changefreq>daily</changefreq><priority>0.50</priority></url>
</urlset>
These are the steps to get Java objects in your code representing the above XML.
1. Download Castor from here
2. Create an XSD file for the above XML (there are online schema generator like HIT software
<?xml version="1.0" encoding="utf-8" ?>
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema">
<xs:element name="changefreq">
<xs:complexType mixed="true" />
</xs:element>
<xs:element name="lastmod">
<xs:complexType mixed="true" />
</xs:element>
<xs:element name="loc">
<xs:complexType mixed="true" />
</xs:element>
<xs:element name="priority">
<xs:complexType mixed="true" />
</xs:element>
<xs:element name="url">
<xs:complexType>
<xs:sequence>
<xs:element ref="loc" />
<xs:element ref="lastmod" />
<xs:element ref="changefreq" />
<xs:element ref="priority" />
</xs:sequence>
</xs:complexType>
</xs:element>
<xs:element name="urlset">
<xs:complexType>
<xs:sequence>
<xs:element ref="url" maxOccurs="unbounded" />
</xs:sequence>
<xs:attribute name="xsi:schemaLocation" type="xs:string" />
</xs:complexType>
</xs:element>
</xs:schema>
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema">
<xs:element name="changefreq">
<xs:complexType mixed="true" />
</xs:element>
<xs:element name="lastmod">
<xs:complexType mixed="true" />
</xs:element>
<xs:element name="loc">
<xs:complexType mixed="true" />
</xs:element>
<xs:element name="priority">
<xs:complexType mixed="true" />
</xs:element>
<xs:element name="url">
<xs:complexType>
<xs:sequence>
<xs:element ref="loc" />
<xs:element ref="lastmod" />
<xs:element ref="changefreq" />
<xs:element ref="priority" />
</xs:sequence>
</xs:complexType>
</xs:element>
<xs:element name="urlset">
<xs:complexType>
<xs:sequence>
<xs:element ref="url" maxOccurs="unbounded" />
</xs:sequence>
<xs:attribute name="xsi:schemaLocation" type="xs:string" />
</xs:complexType>
</xs:element>
</xs:schema>
3. Generate Java objects from the schema using an ANT task
4. Write a simple test case to test the code generation:
FileReader reader = new FileReader(Settings.getInstance("sitemap"));
Urlset urls = Urlset.unmarshal(reader); assertNotNull(urls);
assertEquals(urls.getUrlCount(), 35);
for(int i = 0; i <>
org.castor.sitemap.Url theUrl = urls.getUrl(i);
String currentUrl = theUrl.getLoc().getContent();
System.out.print(currentUrl); assertNotNull(currentUrl);
}
}
P.S. I had a problem with a required attribute. It seems Castor was not mapping correctly xsi:schemaLocation attribute. In my case I modified the generated XSD, still looking at this issue.
Wednesday, March 25, 2009
Changing GlassFish Java JDK version
Following the previous post, if one wants to update GlassFish JDK's version has to do the following:
Goto the config folder, edit the asenv.bat file and change the following to the desired version:
set AS_JAVA=D:\java\jdk1.6.0_11\jre/..
Goto the config folder, edit the asenv.bat file and change the following to the desired version:
set AS_JAVA=D:\java\jdk1.6.0_11\jre/..
VisualVM installation problem
VisualVM is a visual tool integrating several commandline JDK tools and lightweight profiling capabilities. Designed for both production and development time use, it further enhances the capability of monitoring and performance analysis for the Java SE platform.
One can download it here
One can use it to monitor glassfish. Here is a good blog how to configure the glassfish plugin.
I had an issue with the installation. The issue was that I was running JDK6.12
Switching to JDK6.11 solved the problem.
One can download it here
One can use it to monitor glassfish. Here is a good blog how to configure the glassfish plugin.
I had an issue with the installation. The issue was that I was running JDK6.12
Switching to JDK6.11 solved the problem.
Monday, March 9, 2009
Enabling GZIP on GlassFish on v2
I had problems enabling GZIP on GlassFish on V2. For later version there are new settings like
compression = force
But the following settings worked for me at least.
One can configure GZIP from the GUI for the http-listener nodes as follows:

Using FireBug (or Fiddler) one can verify that the request is GZIPped as the following diagram shows.
compression = force
But the following settings worked for me at least.
One can configure GZIP from the GUI for the http-listener nodes as follows:
Using FireBug (or Fiddler) one can verify that the request is GZIPped as the following diagram shows.
Sunday, February 15, 2009
GlassFish and Java memory allocation
Last week I was very confused and bewildered when all of a sudden I could no longer find my compiled JSPs.
We are using GlassFish application server and normally one can find the compiled JSPs in the generated folder.
Anyway after the amusement passed I realised that something must have changed and remembered one of my mottos "Remember the solution!"
Basically what happened was that the server Java JDK was upgraded to 1.6
One of the features of 1.6 is memory allocation. Thus JSPs are compiled and stored in memory. The benefits are obvious, performance! That's where the JSPs have gone!
Anyway, GlassFish has a setting, keepGenerated. Prior version 1.6 this setting is set to true. In 1.6 the default is false. If you are using 1.6 and want to debug the compiled JSPs change keepgenerated (found in default-web.xml and sun-web.xml) to false.
Remember the solution!
We are using GlassFish application server and normally one can find the compiled JSPs in the generated folder.
Anyway after the amusement passed I realised that something must have changed and remembered one of my mottos "Remember the solution!"
Basically what happened was that the server Java JDK was upgraded to 1.6
One of the features of 1.6 is memory allocation. Thus JSPs are compiled and stored in memory. The benefits are obvious, performance! That's where the JSPs have gone!
Anyway, GlassFish has a setting, keepGenerated. Prior version 1.6 this setting is set to true. In 1.6 the default is false. If you are using 1.6 and want to debug the compiled JSPs change keepgenerated (found in default-web.xml and sun-web.xml) to false.
Remember the solution!
Friday, February 13, 2009
Selenium and https
Selenium is a great tool to automate tests for your website. Unfortunately there is a glitch when some of the pages redirect to https.
Selenium can't accept a certificate and when running FireFox, FireFox runs with a new clean profile so the exception to the https site is lost.
A way around this problem is to create a "selenium profile" and when running Selenium Server one specifies the custom profile to be used, thus the exception will be in the profile and the tests will pass!
Here is a breakdown of the steps involved to get it running:
1. Close down instances of FireFox
2. Run FireFox from command line as follows: C:\Program Files\Mozilla Firefox\firefox.exe -ProfileManager
3. Profile dialog loads up, create a new profile. I called it SeleniumProfile and added a new folder in my selenium test suite, something as follows: /mysuite/seleniumprofile
4. Browse to the HTTPS page (with self-signed certificate) and accept the self-signed certificate when prompted. An exception is added in the profil
5. Close the browser.
6. Add the profile to the Selenium Server. The command will look something as follows:
java -jar selenium-server.jar -firefoxProfileTemplate "C:\mysuite\seleniumprofile"
That's it, kick in your lovely automated tests and let them roll without https problems!
Selenium can't accept a certificate and when running FireFox, FireFox runs with a new clean profile so the exception to the https site is lost.
A way around this problem is to create a "selenium profile" and when running Selenium Server one specifies the custom profile to be used, thus the exception will be in the profile and the tests will pass!
Here is a breakdown of the steps involved to get it running:
1. Close down instances of FireFox
2. Run FireFox from command line as follows: C:\Program Files\Mozilla Firefox\firefox.exe -ProfileManager
3. Profile dialog loads up, create a new profile. I called it SeleniumProfile and added a new folder in my selenium test suite, something as follows: /mysuite/seleniumprofile
4. Browse to the HTTPS page (with self-signed certificate) and accept the self-signed certificate when prompted. An exception is added in the profil
5. Close the browser.
6. Add the profile to the Selenium Server. The command will look something as follows:
java -jar selenium-server.jar -firefoxProfileTemplate "C:\mysuite\seleniumprofile"
That's it, kick in your lovely automated tests and let them roll without https problems!
Tuesday, January 20, 2009
Firefox plugins
Firefox is de facto browser for web developers. There are plenty of plugins that makes life easier to develop and debug DOM.
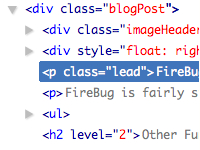
Firebug is the best add-on to debug your DOM structure, modify CSS on the fly...
If one is working with mobile and wants to change the user agent the add-on User Agent Switcher is great.
Want to view how your page looks in IE within FireFox here is an add-on to use IE NetRenderer
Want to check your pageweight and load time of pages you can use the following add-on Hammerhead. Alternatively one can use YSlow although personally I find it inconsistent.
For (X)Html validation here are some add-ons:
HTML Validator
Total Validator
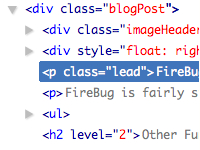
Firebug is the best add-on to debug your DOM structure, modify CSS on the fly...
If one is working with mobile and wants to change the user agent the add-on User Agent Switcher is great.
Want to view how your page looks in IE within FireFox here is an add-on to use IE NetRenderer
Want to check your pageweight and load time of pages you can use the following add-on Hammerhead. Alternatively one can use YSlow although personally I find it inconsistent.
For (X)Html validation here are some add-ons:
HTML Validator
Total Validator
Monday, January 19, 2009
JQuery and more...
JQuery is great to write RIAs (Rich Interface Applications). Its light and one can do everything from handling easily HTML to AJAX.
Add to that some great plugins out there and one can easily write RIAs.
Here a couple of good links for plugins:
http://www.smashingmagazine.com/2009/01/15/45-new-jquery-techniques-for-a-good-user-experience/
http://www.noupe.com/ajax/50-amazing-jquery-examples-part1.html
Also, if you are planning to build a CMS using AJAX this is a great library to use
http://extjs.com/
Its a very powerful library here are some samples of its capabilities:
http://extjs.com/deploy/dev/examples/samples.html
Recently it was announced that Microsoft and Nokia will be adopting JQuery. In particular, JQuery will be bundled within Visual Studio.
Add to that some great plugins out there and one can easily write RIAs.
Here a couple of good links for plugins:
http://www.smashingmagazine.com/2009/01/15/45-new-jquery-techniques-for-a-good-user-experience/
http://www.noupe.com/ajax/50-amazing-jquery-examples-part1.html
Also, if you are planning to build a CMS using AJAX this is a great library to use
http://extjs.com/
Its a very powerful library here are some samples of its capabilities:
http://extjs.com/deploy/dev/examples/samples.html
Recently it was announced that Microsoft and Nokia will be adopting JQuery. In particular, JQuery will be bundled within Visual Studio.
Subscribe to:
Posts (Atom)